Text View
xib-based
Adding NSTextView control to xib interface, change its custom class to component class name of your exported.
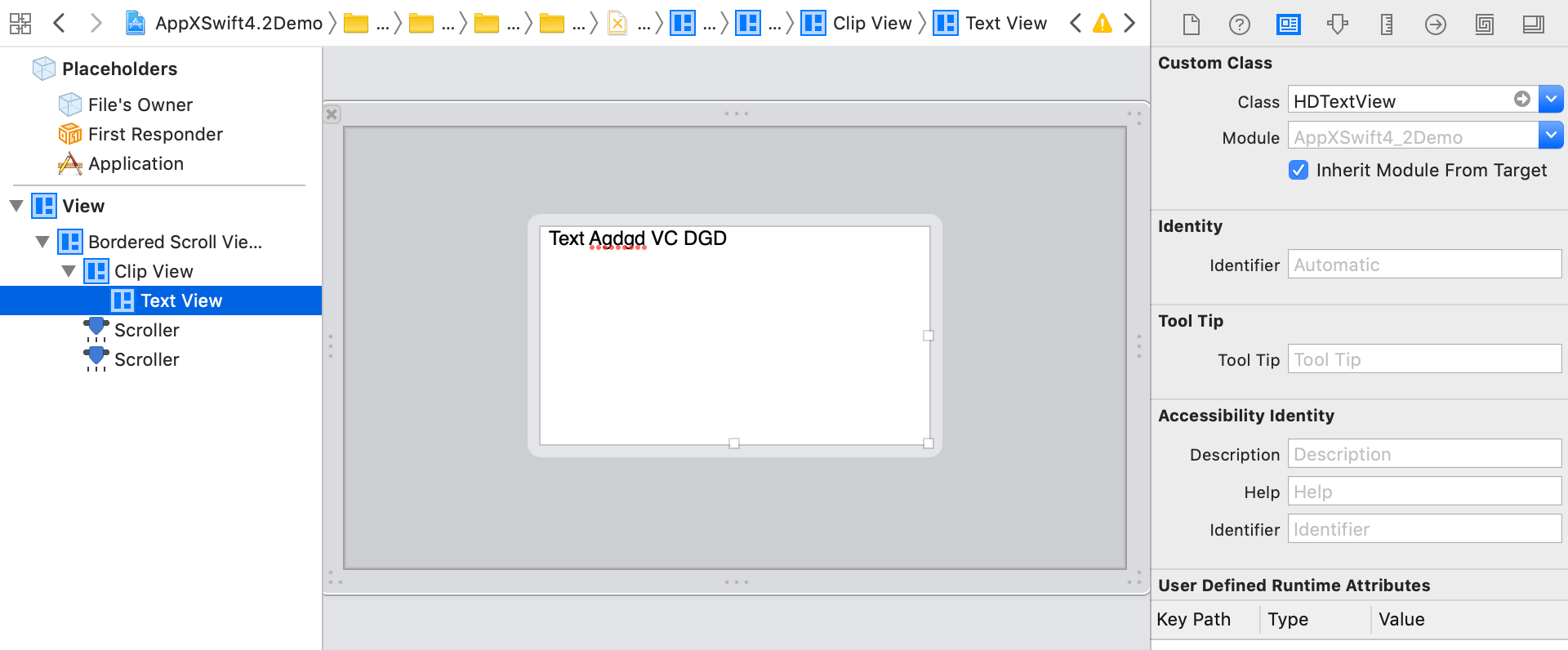
code-based
Declare lazy load variable in the controller class and add it to the view.
import Cocoa
class ViewController: NSViewController {
lazy var textView: HDTextView = {
let textView = HDTextView()
textView.isVerticallyResizable = true
textView.isHorizontallyResizable = false
textView.autoresizingMask = .width
textView.textContainer?.widthTracksTextView = true
textView.isEditable = true
return textView
}()
lazy var scrollView: NSScrollView = {
let scrollView = NSScrollView(frame: NSRect(x: 100, y: 100, width: 80, height: 80))
scrollView.hasVerticalScroller = true
scrollView.hasHorizontalScroller = false
scrollView.focusRingType = .none
scrollView.autohidesScrollers = true
scrollView.borderType = .noBorder
scrollView.autoresizingMask = [.width , .height]
return scrollView
}()
override func viewDidLoad() {
super.viewDidLoad()
scrollView.documentView = textView
textView.frame = NSRect(origin: NSZeroPoint, size: scrollView.contentSize)
self.view.addSubview(scrollView)
}
}